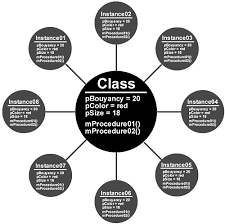
Hi friends this is the stuff for Object oriented programming concepts and the OOAD concepts based on previous year papers and the various faq's. Hope this will help in your placements...
OOPS
1. Name some pure object oriented languages.
Ø Smalltalk,
Ø Java,
Ø Eiffel,
Ø Sather.
2. What do you mean by the words ‗Abstraction‘, ‗Separation‘, ‘Composition‘, and ‗Generalization‘?
Abstraction:
Simplifying the description of a real world entity to its essentials.
Separation:
Treating what an entity does and how it does it independently of each other.
Composition:
Building complex whole components by assembling simpler parts in one of the two ways, Association and aggregation.
Generalization:
Identifying common elements in an entity.
3. What is information hiding?
Information hiding is a mechanism that separates the implementation of the class from its user.
4. Differentiate between the message and method.
Message Method
Objects communicate by sending messages Provides response to a message.
to each other.
A message is sent to invoke a method. It is an implementation of an
operation.
5. What is the interface of a class?
The interface of the class is the view provided to the outside world, which hides its internal structure and behaviour.
6. What is an adaptor class or Wrapper class?
A class that has no functionality of its own. Its member functions hide the use of a third party software component or an object with the non-compatible interface or a non- object- oriented implementation.
7. What is a node class?
A node class is a class that,
Ø relies on the base class for services and implementation,
Ø provides a wider interface to te users than its base class,
Ø relies primarily on virtual functions in its public interface
Ø depends on all its direct and indirect base class
Ø can be understood only in the context of the base class
Ø can be used as base for further derivation
Ø can be used to create objects.
A node class is a class that has added new services or functionality beyond the services inherited from its base class.
8. What is an orthogonal base class?
If two base classes have no overlapping methods or data they are said to be independent of, or orthogonal to each other. Orthogonal in the sense means that two classes operate in different dimensions and do not interfere with each other in any way. The same derived class may inherit such classes with no difficulty.
9. What is a container class? What are the types of container classes?
A container class is a class that is used to hold objects in memory or external storage. A container class acts as a generic holder. A container class has a predefined behavior and a well-known interface. A container class is a supporting class whose purpose is to hide the topology used for maintaining the list of objects in memory. When a container class contains a group of mixed objects, the container is called a heterogeneous container; when the container is holding a group of objects that are all the same, the container is called a homogeneous container.
10. What is a protocol class?
An abstract class is a protocol class if:
Ø it neither contains nor inherits from classes that contain member data, non-virtual functions, or private (or protected) members of any kind.
Ø it has a non-inline virtual destructor defined with an empty implementation,
Ø all member functions other than the destructor including inherited functions, are declared pure virtual functions and left undefined.
11. What is a mixin class?
A class that provides some but not all of the implementation for a virtual base class is often called mixin. Derivation done just for the purpose of redefining the virtual functions in the base classes is often called mixin inheritance. Mixin classes typically don't share common bases.
12. What is a concrete class?
A concrete class is used to define a useful object that can be instantiated as an automatic variable on the program stack. The implementation of a concrete class is defined. The concrete class is not intended to be a base class and no attempt to minimize dependency on other classes in the implementation or behavior of the class.
13. What is the handle class?
A handle is a class that maintains a pointer to an object that is programmatically accessible through the public interface of the handle class.
In case of abstract classes, unless one manipulates the objects of these classes through pointers and references, the benefits of the virtual functions are lost. User code may become dependent on details of implementation classes because an abstract type cannot be allocated statistically or on the stack without its size being known. Using pointers or references implies that the burden of memory management falls on the user. Another limitation of abstract class object is of fixed size. Classes however are used to represent concepts that require varying amounts of storage to implement them.
A popular technique for dealing with these issues is to separate what is used as a single object in two parts: a handle providing the user interface and a representation holding all or most of the object's state. The connection between the handle and the representation is typically a pointer in the handle. Often, handles have a bit more data than the simple representation pointer, but not much more. Hence the layout of the handle is typically stable, even when the representation changes and also that handles are small enough to move around relatively freely so that the user needn‘t use the pointers and the references.
14. What is an action class?
The simplest and most obvious way to specify an action in C++ is to write a function. However, if the action has to be delayed, has to be transmitted 'elsewhere' before being performed, requires its own data, has to be combined with other actions, etc then it often becomes attractive to provide the action in the form of a class that can execute the desired action and provide other services as well. Manipulators used with iostreams is an obvious example.
A common form of action class is a simple class containing just one virtual function.
class Action{
public:
virtual int do_it( int )=0;
virtual ~Action( );
}
Given this, we can write code say a member that can store actions for later execution without using pointers to functions, without knowing anything about the objects involved, and without even knowing the name of the operation it invokes. For example:
class write_file : public Action{
File& f;
public:
int do_it(int){
return fwrite( ).suceed( );
}
};
class error_message: public Action{
response_box db(message.cstr( ),"Continue","Cancel","Retry");
switch (db.getresponse( )) {
case 0: return 0;
case 1: abort();
case 2: current_operation.redo( );return 1;
}
};
A user of the Action class will be completely isolated from any knowledge of derived classes such as write_file and error_message.
15. What are seed classes?
In C++, you design classes to fulfill certain goals. Usually you start with a sketchy idea of class requirements, filling in more and more details as the project matures. Often you wind up with two classes that have certain similarities. To avoid duplicating code in these classes, you should split up the classes at this point, relegating the common features to a parent and making separate derived classes for the different parts. Classes that are made only for the purpose of sharing code in derived classes are called seed classes.
16. What is an accessor?
An accessor is a class operation that does not modify the state of an object. The accessor functions need to be declared as const operations
17. What is an inspector?
Messages that return the value of an attribute are called inspector.
18. What is a modifier?
A modifier, also called a modifying function is a member function that changes the value of at least one data member. In other words, an operation that modifies the state of an object. Modifiers are also known as ‗mutators‘.
19. What is a predicate?
A predicate is a function that returns a bool value.
20. What is a facilitator?
A facilitator causes an object to perform some action or service.
21. State the "Rule of minimality" and its corollary?
The rule of minimality states that unless a behavior is needed, it shouldn't be part of the ADT.
Corollary of the rule of minimality: If the function or operator can be defined such that, it is not a member. This practice makes a non-member function or operator generally independent of changes to the class's implementation.
22. What is reflexive association?
The 'is-a' is called a reflexive association because the reflexive association permits classes to bear the is-a association not only with their super-classes but also with themselves. It differs from a 'specializes-from' as 'specializes-from' is usually used to describe the association between a super-class and a sub-class. For example:
Printer is-a printer.
23. What is slicing?
Slicing means that the data added by a subclass are discarded when an object of the subclass is passed or returned by value or from a function expecting a base class object.
Consider the following class declaration:
class base{
...
base& operator =(const base&);
base (const base&);
}
void fun( ){
base e=m;
e=m;
}
As base copy functions don't know anything about the derived only the base part of the derived is copied. This is commonly referred to as slicing. One reason to pass objects of classes in a hierarchy is to avoid slicing. Other reasons are to preserve polymorphic behavior and to gain efficiency.
24. What is a Null object?
It is an object of some class whose purpose is to indicate that a real object of that class does not exist. One common use for a null object is a return value from a member function that is supposed to return an object with some specified properties but cannot find such an object.
25. Define precondition and post-condition to a member function.
Precondition:
A precondition is a condition that must be true on entry to a member function. A class is used correctly if preconditions are never false. An operation is not responsible for doing anything sensible if its precondition fails to hold.
For example, the interface invariants of stack class say nothing about pushing yet another element on a stack that is already full. We say that isful() is a precondition of the push operation.
Post-condition:
A post-condition is a condition that must be true on exit from a member function if the precondition was valid on entry to that function. A class is implemented correctly if post-conditions are never false.
For example, after pushing an element on the stack, we know that isempty() must necessarily hold. This is a post-condition of the push operation.
26. What is class invariant?
A class invariant is a condition that defines all valid states for an object. It is a logical condition to ensure the correct working of a class. Class invariants must hold when an object is created, and they must be preserved under all operations of the class. In particular all class invariants are both preconditions and post-conditions for all operations or member functions of the class.
27. What are the conditions that have to be met for a condition to be an invariant of the class?
Ø The condition should hold at the end of every constructor.
Ø The condition should hold at the end of every mutator(non-const) operation.
28. What are proxy objects?
Objects that points to other objects are called proxy objects or surrogates. Its an object that provides the same interface as its server object but does not have any functionality. During a method invocation, it routes data to the true server object and sends back the return value to the object. template
public:
class Array1D{
public:
T& operator[] (int index);
const T& operator[] (int index) const;
...
};
Array1D operator[] (int index);
const Array1D operator[] (int index) const;
...
};
The following then becomes legal:
Array2D
........
cout<B, B=>c then A=>c.
A. Salesman, B. Employee, C. Person.
Note:
All the other relationships satisfy all the properties like Structural properties, Interface properties, Behaviour properties.
12. Differentiate Aggregation and containment?
Aggregation is the relationship between the whole and a part. We can add/subtract some properties in the part (slave) side. It won't affect the whole part.
Best example is Car, which contains the wheels and some extra parts. Even though the parts are not there we can call it as car.
But, in the case of containment the whole part is affected when the part within that got affected. The human body is an apt example for this relationship. When the whole body dies the parts (heart etc) are died.
13. Can link and Association applied interchangeably?
No, You cannot apply the link and Association interchangeably. Since link is used represent the relationship between the two objects.
But Association is used represent the relationship between the two classes.
14. List out some of the object-oriented methodologies.
Ø Object Oriented Development (OOD) (Booch 1991,1994).
Ø Object Oriented Analysis and Design (OOA/D) (Coad and Yourdon 1991).
Ø Object Modelling Techniques (OMT) (Rumbaugh 1991).
Ø Object Oriented Software Engineering (Objectory) (Jacobson 1992).
Ø Object Oriented Analysis (OO (Shlaer and Mellor 1992).
Ø The Fusion Method (Coleman 1991).
15. What is meant by "method-wars"?
Before 1994 there were different methodologies like Rumbaugh, Booch, Jacobson, Meyer etc who followed their own notations to model the systems. The developers were in a dilemma to choose the method which best accomplishes their needs. This particular time-span was called as "method-wars".
16. Whether unified method and unified modeling language are same or different?
Unified method is convergence of the Rumbaugh and Booch. Unified modeling lang. is the fusion of Rumbaugh, Booch and Jacobson as well as Betrand Meyer (whose contribution is "sequence diagram"). Its' the superset of all the methodologies.
17. Who were the three famous amigos and what was their contribution to the object community?
The Three amigos namely,
Ø James Rumbaugh (OMT): A veteran in analysis who came up with an idea about the objects and their Relationships (in particular Associations).
Ø Grady Booch: A veteran in design who came up with an idea about partitioning of systems into subsystems.
Ø Ivar Jacobson (Objectory): The father of USECASES, who described about the user and system interaction.
17. Differentiate the class representation of Booch,Rumbaugh and UML?
If you look at the class representaiton of Rumbaugh and UML, It is some what similar and both are very easy to draw.
Representation:
OMT
ClassName
+Public Attribute;#protected Attribute;-private Attribute;
+Public Method();#Protected Method();-private Method();
UML.
ClassName<
+Public Attribute;#protected Attribute;-private Attribute;classattribute;
+Public Method();#Protected Method();-private Method();classmethod();
Booch:
In this method classes are represented as "Clouds" which are not very easy to draw as for as the developer's view is concern.
Representation:
18. What is an USECASE?why it is needed?
A Use Case is a description of a set of sequence of actions that a system
performs that yields an observable rsult of value to a particular action.
Simply, in SSAD process <=> In OOAD USECASE. It is represented elliptically.
Representation:
19. Who is an Actor?
An Actor is someone or something that must interact with the system.In addition to that an Actor initiates the process (that is USECASE).
It is represesnted as a stickman like this.
Representation:
20. What is guard condition?
Guard condition is one which acts as a firewall. The access from a particular object can be made only when the particular condition is met.
For Example,
here the object on the customer acccess the ATM facility only when the guard condition is met.
21. Differentiate the following notations?
I:
II:
In the above I represention Student Class sends message to Course Class
but in the case of second , the data is transfered from student Class to Course Class
22. USECASE is an implementaion independent notation. How will the designer give the implementaion details of a particular USECASE to the programmer?
This can be accompllished by specifying the relationship called "refinement" that talkes about the two different abstraction of the same thing.
For example,
In the above example calculate Pay is an USECASE. It is refined in terms of giving the implementation details. This kind of connection is related by means of ―refinement‖.
23. Suppose a class acts an Actor in the problem domain,how can i represent it in the
static model?
In this senario you can use ―stereotype‖.since stereotype is just a string that gives extra semantic to the particular entity/model element.
It is given with in the << >>.
Class<< Actor>>
Attributes
MemberFunctions
24. Why does the function arguments are called as "signatures"?
The arguments distinguishes functions with the same name (functional polymorphism). The name alone does not necessarily identify a unique function. However, the name and its arguments (signatures) will uniquely identify a function.
In real life we see suppose,in class there are two guys with same name.but they can be easily identified by their signatures.The same concept is applied here.
For example:
class person
{
public:
char getsex();
void setsex(char);
void setsex(int);
};
In this example we can see that there is a function setsex() with same name but with different signature.
0 comments:
Post a Comment