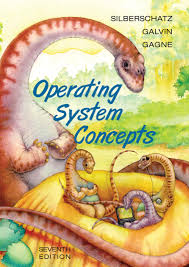
Pages>>
1 2 3 4 5 6 7 8Here we are giving chapter wise notes of operating system based on operating system concepts by galvin
MEMORY
q) on what do scheme for
memory management depends?
a) hardware.
note:- memory can be viewed as the large array of bytes , each having an
address.
q) what is the input queue?
a) collection of processes on the disk , waiting to be brought into the memory for execution , forms the input queue.
note:- compiler maps the symbolic address of the program into the relocatable address.loader convert it into physical address
q) different types of binding of instructions and data to
memory address?
a) 1) compile time:- if we know at compile time where the program will reside in memory.if location changes, we need to
recompile it. eg:- MS-DOS .COM -format programs use this technique.
2) load time:-
3) execution time:- if process is to be moved in memory during execution time,run time binding is used.
q) what is logical address
space and the physical address space?
a) address generated by cpu is logical address and address seen by memory is physical address. set of addresses is called
address space.
note:- in case of compile time and load time binding , the logical address and physical address are the same, whereas in
run time they are not.
q) what is
memory management unit?
a) run time logical to physical address mapping is done by MMU.
q) what is concept of dynamic loading?
a) here the routinue is not loaded into memory until it is called.only main program is loaded.after call, routinue is loaded
and program's address table is also updated.to take advantage of this concept, programmer has to take proper care,not the
responsibilty of the O.S.
q) what is there in linking?
a) here the other modules and system libraries are included into the program image.
q) what is
dynamic linking?
a) here all the system libraries or atleast the routines referenced in them are linked only when call is made at run time.
q) what is a stub?
a) it is used to support the dynamic linking.it is included for each library routine reference.it is a small piece of code,
that tells how to locate the library-routine in memory or how to load if routine not present.
** its advantage is that all the processes use only 1 copy of the routine.
q) what is concept of overlays?
a) it enables the process to be larger than the memory allocated to it. only required instructions and data are to be kept in the
memory. when other instructions are needed , they are brought in place of previous data, no longer needed.
eg:- 2 pass assembler.
note:- an overlay driver is used that reads code into memory.it also is responsibility of programmer to take advantage of
this concept.programmer only designs the overlay, o.s only do I/O here.
q) what is swapping?
a) it is process of shifting a process to backing store(swap out), and bringing a process to memory(swap in).
q) what is rollin and roll out?
a) it is variant of swapin and swap out. it is done when a higher priority process wants to come into the memory.
q) do a process swapped out , needs to be swapped in , in the same location?
a) in case of compiletime and loadtime binding---yes, but not in case of run time address binding.
q) when a process can't be swapped out?
a) if it's not idle.if a process is waiting for the I/O ,and I/O is asynchronously accessing the user memory for I/O buffers,
then it can't be swapped out.
**** in case of contigous memory allocation:-
note:- memory divided into 2 parts:-
1) o.s 2) user programs.
q) how to provide memory protection?
a) using a relocation and limit register.
note:- logical address is first compared with the limit register.
q) what is transient operating -system code?
a) o.s contains code and buffer space for devicedrivers. if device driver not commonly used,no need to keep the code and
data in memeory. such code that comes and goes as needed is called transient o.s.
q) what is MFT?
a) it is multiprogramming with fixed number of tasks. here the memory is divided into fixed sized of partitions. in 1
partition 1 process can reside at a time.it was used by IBM
OS/360, now not in use.
q) what is MVT?
a) it is multiprogramming with variable number of tasks.primarily used in batch environment.
q) how MVT is implemented?
a) OS maintains a table indicating which parts of memory are free and which are allocated.
q) what is a hole?
a) free part of memory is called a hole.
q) how dynamic storage allocation problem solved?
a) it relates to how to satisfy the request of size n from the available holes.many strategies are there:- first fit, best
fit,worst fit.
q) what is the disadvantage of these algorithms above?
a) they suffer from external fragmentation.
q) what is external fragmentation?
a) it exists when we have total free memory to accomodate a process, but it is not contiguous.
q) what is
internal fragmentation?
a) when
physical memory is broken into fixed sized partitions.here the process may be smaller than the size of the partition.
so rest of space in partition is wasted. it is called internal fragmentation.
q) what is compaction?
a) it is one of the solution to the external fragmentation.here the free memory is moved to one part and all pragrams and
data on other side. this can be done if address binding is done at run time. it involves a lot of cost also.
note:- other solution to external fragmentation is
paging and segmantation(here program need not to be in contiguous memory)
q) what is paging?
a) it allows the physical address space of the process to be non-contiguous. earlier when processes used to be swapped out
we need to search for free space on disk(backing store) too. so, external fragmantation on disk also occured.
q) concept of paging?
a) physical memory is broken into fixed sise blocks called frames.logical memory is broken into pages of same size of frames.
backing store is also divided into the blocks of size of frames.
when a process is to be loaded , its pages must be loaded into the frames.
q) now how cpu generates address?
a) now address has 2 parts:- p and d. p is the index into the
page table, from where we get the frame number, which combines
with d part to form physical address.
q) how to decide how many bits for p and d?
a) page size is defined by hardware.this size is generally the power of 2.if size of logical address space is pow(2,m) and
the page size is pow(2,n), then higher order m-n bits will tell the page number and the n lower order bits will tell the
page offset.
note:- in paging we dont have external fragmentation, but we do have internal fragmentation.
q) so, can we have small page size to reduce the internal fragmentation?
a) but then the page table will grow in size , and overhead to maintian will increase.also with smaller page size disk I/O
will not be efficient.
note:- when a process arrives in the system , its size in pages is expressed.
q) what is frame table?
a) it is a table maintained by the O.s, which has 1 entry for each frame of physical memory.it tells whether a frame is free
or not, if not, then to which process it is allocated.
note:- a system call is made,suppose for I/O, then buffer address is passed as the parameter.
note:- for each process there is a page table.
q) do paging increase context switch time?
a) yes, because dispatcher has also to define hardware page table.
note:- the page table pointer is also stored in the process control block.
q) how to implement page table?
a) way 1) use of dedicated set of registers:- dispatcher needs to load these registers , in context switch.
this method is not feasible if page table has millions of entries.
way 2) here we store the page table in the
main memory and PTBR stores its address.here context switch time is less
but here to make a access in memory , 2 memory accesses are required.
q) what is
TLB(translation look-aside buffer)?
a) it is a high speed associative memory, with 2 fields tag and value.upto 60 to 1024 entries it can handle.it will contain
only few of the page table entries.
q) what if a search in TLB makes a miss?
a) then the reference to memory page table is made.the new entry is also added to the TLB. if no entry free, 1 need to be
replaced.
q) what do u mean that some entries in TLB are wired down?
a) it means that they cannot be removed from there.entries for kernel code are wired down.
q) what are protection bits?
a) they are stored into the page table only and they indicate during address mapping, that the particular page is read-write
or read-only.
q) what is valid-invalid bit?
a) it is 1 more bit attached to the page table entry.valid bit indicates that this page is in process's logical address space
and thus legal, and invalid bit indicates that the page is not in the logical address space of the process.they cause trap to
O.S.
note:- advantage of paging is sharing common code.it can be done by setting entry in the page table.
q) what is reentrant code?
a) that never changes during the execution. eg:- code of a text editor.it can be executed by more processes at same time.
q) name some programs that can be shared?
a) compilers,database-systems, run-time libraraies,etc. code shared must be reentrant.
q) what is segmentation?
a) here the user view of memory is as the collection of various segments.each segment has a name and length.the address here
consists of the segment number and the offset.
.
note:- when a user program is compiled, the compiler itself constructs various segments.
q) who does mapping here?
a) segmenation table, each entry here has base and limit of segment. here logical address has 2 parts , segment number and
offset, offset is compared against the segment limit.
q) what are advantages of segmentation?
a)1) main, functions,procedures, global variables,stacks,arrays,tables etc are various segments.because of segment containing
entries of same type,protection can be easily provided.
2) sharng of code and data can be done. only need to change process's segment table.
note:- in case of segmentation, the care must be taken while sharing the code segment, as they refer to themselves. all
sharing processes need to have same segment number for that code segment. this problem is not there in case of read only
segments.Pages>>
1 2 3 4 5 6 7 8